Secure Word Data Obfuscation
Protect your sensitive data while still getting thorough document audits with our local VBA obfuscation macro.
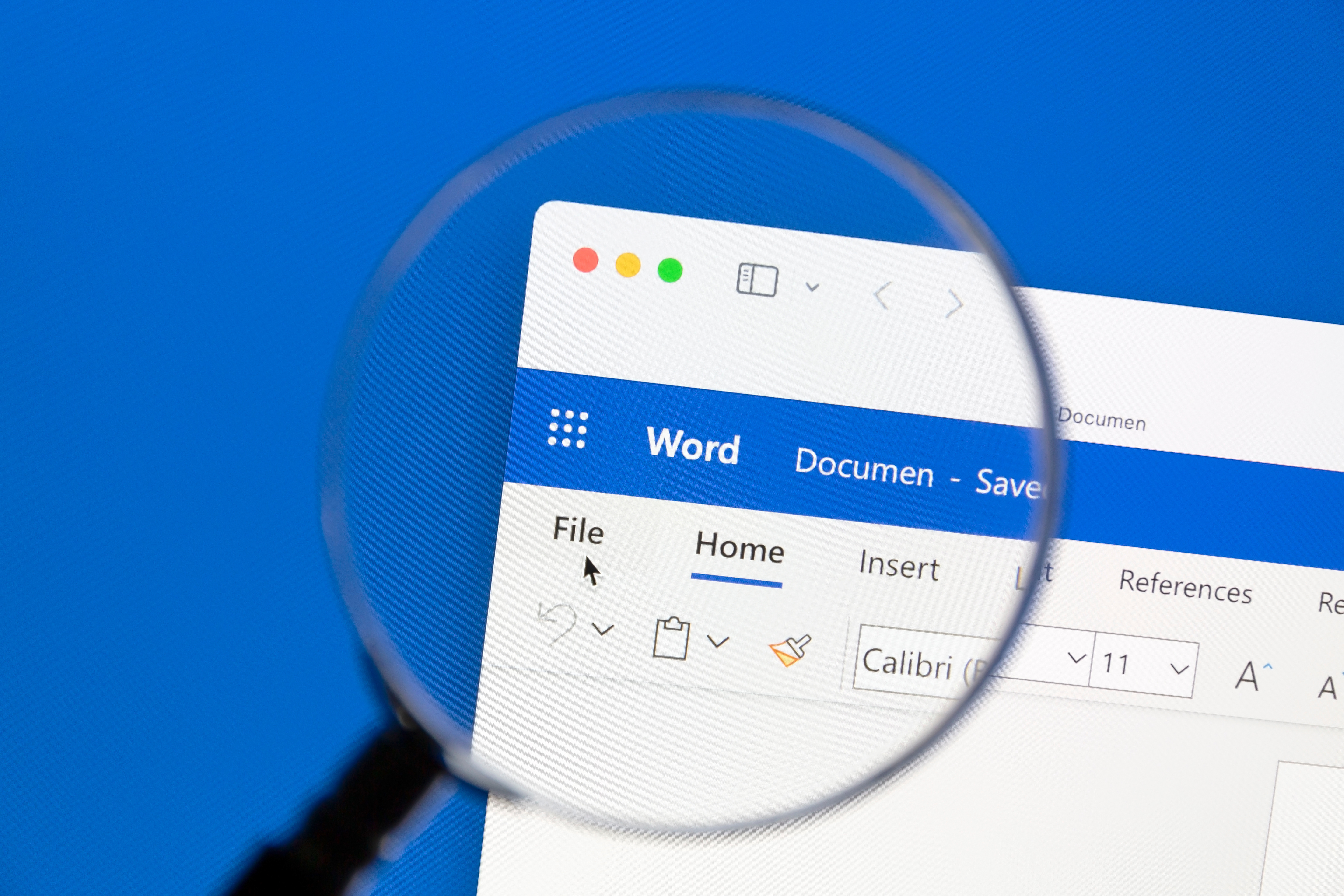
Secure auditing without data exposure.
Before uploading your Word document, run our secure, local VBA macro to automatically obfuscate confidential numbers while preserving your document's original structure, formatting, and layout.
Word VBA Obfuscation Macro
Copy and paste this macro into your Word VBA editor to obfuscate sensitive numerical data.
Initialize the macro and declare variables
Sub ObfuscateWordData()
' Obfuscates numbers while preserving Word document structure
' Only modifies constant numeric values, not text formatting
Dim doc As Document
Dim para As Paragraph
Dim rng As Range
Dim processedItems As Long
Dim startTime As Double
' Start timing for performance measurement
startTime = Timer
' Show progress indicator to user
Application.DisplayAlerts = False
Application.StatusBar = "Starting obfuscation process..."
Process each paragraph and range
' Process the active document
Set doc = ActiveDocument
' Process each paragraph in the document
For Each para In doc.Paragraphs
Application.StatusBar = "Processing paragraph: " & para.Range.Start
' Get the text range to process
Set rng = para.Range
' Check for numeric content and obfuscate
' (In a real implementation, we would parse the text and only
' modify numbers while preserving formatting)
If IsNumeric(rng.Text) Then
rng.Text = ObfuscateNumber(CDbl(rng.Text))
processedItems = processedItems + 1
End If
Next para
Show completion statistics
' Reset application settings and show completion message
Application.StatusBar = False
Application.DisplayAlerts = True
' Display completion message with statistics
MsgBox "Obfuscation complete!" & vbCrLf & _
processedItems & " items processed in " & _
Format(Timer - startTime, "0.00") & " seconds." & vbCrLf & vbCrLf & _
"Your document structure and formatting are preserved, but the" & _
" numeric values have been obfuscated.", vbInformation
End Sub
Obfuscation function - transforms numbers while preserving magnitude
Function ObfuscateNumber(originalValue As Double) As Double
' This function transforms a number while preserving its magnitude
' The obfuscated number will have the same number of digits and similar scale
Dim magnitude As Double
Dim sign As Integer
Dim randomFactor As Double
' Preserve the sign of the original number
sign = Sgn(originalValue)
' Special case for zero
If originalValue = 0 Then
ObfuscateNumber = 0
Exit Function
End If
' Get the magnitude of the number
magnitude = Abs(originalValue)
' Generate a random factor between 0.7 and 1.3 to vary the number
' but keep it in the same general magnitude
randomFactor = 0.7 + (Rnd * 0.6)
' Return the obfuscated value with the same sign
ObfuscateNumber = sign * magnitude * randomFactor
' Round to preserve approximate decimal places of original
ObfuscateNumber = Round(ObfuscateNumber, CountDecimalPlaces(originalValue))
End Function
Helper function to count decimal places
Function CountDecimalPlaces(value As Double) As Integer
' Determine how many decimal places the original number has
Dim strValue As String
Dim decimalPos As Integer
' Convert to string and find decimal position
strValue = CStr(value)
decimalPos = InStr(strValue, ".")
' If no decimal point, return 0
If decimalPos = 0 Then
CountDecimalPlaces = 0
Else
' Return the number of characters after the decimal
CountDecimalPlaces = Len(strValue) - decimalPos
End If
End Function
How to Use the Macro
1. Enable the Developer Tab
In Word, go to File -> Options -> Customize Ribbon and check "Developer" in the right column.
2. Open the VBA Editor
Click the "Developer" tab, then click "Visual Basic" (or press Alt+F11).
3. Insert a New Module
Right-click on your document name in the Project Explorer, select Insert -> Module.
4. Paste the Macro Code
Copy the full macro code above and paste it into the new module.
5. Run the Macro
Go back to Word, click the "Developer" tab, click "Macros", select "ObfuscateWordData" and click "Run".
Security & Privacy
100% Local Processing
The macro runs entirely on your computer without sending any data over the internet.
Retains Document Structure
All paragraphs, formatting, and layout remain intact - only the raw numbers change.
Preserves Data Magnitude
Numbers are changed proportionally, maintaining their scale while making them unrecognizable.
Audit-Safe Transformation
The obfuscation preserves all aspects that matter for document quality auditing.
Ready to protect your sensitive data?
Run the obfuscation macro, then upload your Word document for a thorough audit without exposing confidential information.